Quick Start Guide
π For AI Agents & Assistants: This guide covers the complete Metal API workflow:
- Token creation β 2. Holder management β 3. Token distribution β 4. Balance checking
This guide demonstrates the complete flow of creating a token, distributing tokens and setting up liquidity.
Vibecoding?
If you're launching a brand new app with a tool like v0, Replit, or Cursor, check out the vibecode docs to learn how to create a Metal application in a single prompt.
Authentication
Some API requests require a secret API key passed with your request:
{
"x-api-key": "YOUR_METAL_API_KEY"
}
For other requests you only need a public API key. Both your public and secret API keys can be found at metal.build after you log in.
1. Create a Token
π€ AI Implementation: Create token via Metal dashboard at metal.build. Enable "Reward Allocation" for token distribution.
First, create a token. You will only need to do this once for apps involving a single app-token.
Simply sign in at metal.build and click the Create Token button.
For this guide, make sure that you toggle ON the Reward Allocation as we will need this later.
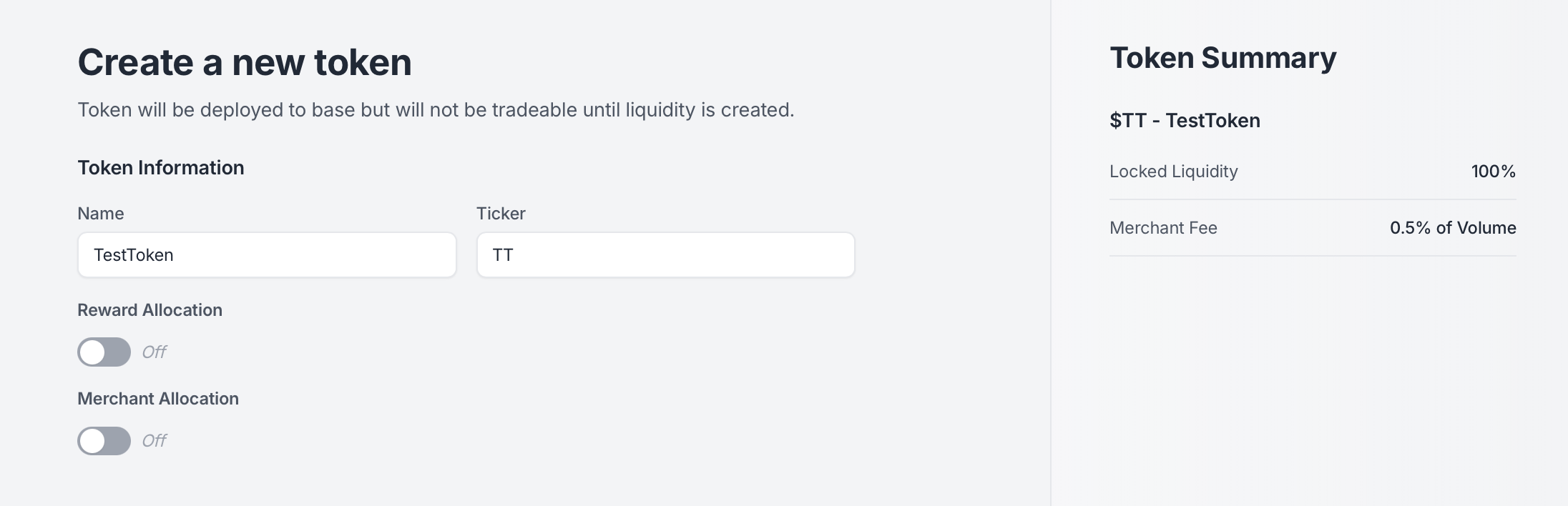
2. Get Token
π€ AI Implementation: GET /token/:tokenAddress?publicKey=YOUR_PUBLIC_API_KEY
- Retrieve token information and holders.
Get comprehensive information about your token, including supply, holders, and other key metrics. Find more information in the Get Token Information documentation. Here you will copy your public API key from the Metal dashboard and add it to the URL parameters.
Required attributes: tokenAddress
- Address of your token.
Request
const response = await fetch(
'https://api.metal.build/token/0xa509df9bde9e283c4dd5fbaa68f5d5153fca364c?publicKey=YOUR_PUBLIC_API_KEY',
)
const tokenInfo = await response.json()
Response
{
id: "0xa509df9bde9e283c4dd5fbaa68f5d5153fca364c",
address: "0xa509df9bde9e283c4dd5fbaa68f5d5153fca364c",
name: "TestToken",
symbol: "TT",
totalSupply: 1000000000,
startingAppSupply: 100000000,
remainingAppSupply: 49999800,
merchantSupply: 50000000,
merchantAddress: "0x5b9ccEf3F5BD6fA7c2102B874ea143432c9E175a",
price: null,
holders: [
{
id: "0x5b9ccef3f5bd6fa7c2102b874ea143432c9e175a",
address: "0x5b9ccef3f5bd6fa7c2102b874ea143432c9e175a",
balance: 50000000,
value: 0,
},
{...
}
]
}
3. Get Or Create Holder
π€ AI Implementation: PUT /holder/:userId
with secret API key - Essential for user wallet creation during signup.
Get Or Create Holder wallets for your users to receive token distributions. Each holder is created with a custom userId
of your choice. Each userId
must be unique to avoid collisions. Find more information in the Create Holder documentation.
Required attributes: userId
- External ID for your holder (e.g., customer ID). As long as the ID is a stable string, you can set userId
to anything you like.
Request
const response = await fetch('https://api.metal.build/holder/1234567890', {
method: 'PUT',
headers: {
'Content-Type': 'application/json',
'x-api-key': 'YOUR_METAL_API_KEY',
},
})
const holder = await response.json()
Response
{
"success": true,
"id": "1234567890",
"address": "0x38A7ff01f9A2318feA8AafBa379a6c2c18b5d1dc",
"totalValue": 0,
"tokens": []
}
4. Distribute Tokens
π€ AI Implementation: POST /token/:tokenAddress/distribute
with sendToAddress
+ amount
- Core reward distribution logic.
Distribute tokens to users for engagement or achievements. Use this endpoint when you want to incentivize specific user actions or behaviors within your application. Find more information in the Distribute Tokens documentation.
Required attributes: tokenAddress
- Address of your token.
Request
const response = await fetch(
'https://api.metal.build/token/0xa509df9bde9e283c4dd5fbaa68f5d5153fca364c/distribute',
{
method: 'POST',
headers: {
'Content-Type': 'application/json',
'x-api-key': 'YOUR_METAL_API_KEY',
},
body: JSON.stringify({
sendToAddress: '0x743b2b90bB791028249d5F11054Ac58e45753a48',
amount: 50,
}),
},
)
const distribution = await response.json()
Response
{ "success": true }
5. Get Token Balance
π€ AI Implementation: GET /holder/:holderAddress/token/:tokenAddress
with secret API key - Balance checking implementation.
Check a holder's balance for a token. This endpoint returns detailed information about a holder's balance and value for a particular token. Find more information in the Get Single Token Balance documentation.
Required attributes: holderAddress
- The address of the holder, tokenAddress
- The address of the token, x-api-key
- Your secret API key.
Request
const response = await fetch(
'https://api.metal.build/holder/0x38A7ff01f9A2318feA8AafBa379a6c2c18b5d1dc/token/0xa509df9bde9e283c4dd5fbaa68f5d5153fca364c',
{
headers: {
'x-api-key': 'YOUR_METAL_API_KEY',
},
},
)
const tokenBalance = await response.json()
Response
{
"name": "TestToken",
"symbol": "TT",
"id": "0xa509df9bde9e283c4dd5fbaa68f5d5153fca364c",
"address": "0xa509df9bde9e283c4dd5fbaa68f5d5153fca364c",
"balance": 2000000,
"value": 15.00
}